Many of us deal or will deal with (connected) M2M/IoT devices. This might be writing firmware for microcontrollers, using a RTOS like
NuttX or a full blown Unix (like) operating system like FreeBSD or Yocto/Poky Linux, creating and building code to run on the device, processing data in the backend or somewhere inbetween. Many of these devices will have sensors to collect data like
GNSS position/time, temperature, light detector, measuring acceleration, see airplanes, detect
lightnings, etc.The backend problem is work but mostly “
solved”. One can rely on something like Amazon IoT or creating a powerful infrastructure using many of the FOSS options for message routing, data storage, indexing and retrieval in C++. In this post I want to focus about the little detail of how data can go from the device to the backend.
To make this thought experiment a bit more real let’s imagine we want to build a bicycle lock/tracker. Many of my colleagues ride their bicycle to work and bikes being stolen remains a big tragedy. So the primary focus of an IoT device would be to prevent theft (make other bikes a more easy target) or making selling a stolen bicycle more difficult (e.g. by easily checking if something has been stolen) and in case it has been stolen to make it more easy to find the current location.
Architecture
Let’s assume two different architectures. One possibility is to have the bicycle actively acquire the position and then try to push this information to a server (“active push”). Another approach is to have fixed installed scanning stations or users to scan/report bicycles (“passive pull”). Both lead to very different designs.
Active Push
The system would need some sort of GNSS module, a microcontroller or some full blown SoC to run Linux, an accelerator meter and maybe more sensors. It should somehow fit into an average bicycle frame, have good antennas to work from inside the frame, last/work for the lifetime of a bicycle and most importantly a way to bridge the air-gap from the bicycle to the server.
 |
Push architecture |
The device would not know its position or if it is moved. It might be a simple barcode/QR code/NFC/iBeacon/etc. In case of a barcode it could be the serial number of the frame and some owner/registration information. In case of NFC it should be a randomized serial number (if possible to increase privacy). Users would need to scan the barcode/QR-code and an application would annotate the found bicycle with the current location (cell towers, wifi networks, WGS 84 coordinate) and upload it to the server. For NFC the smartphone might be able to scan the tag and one can try to put readers at busy locations.
The incentive for the app user is to feel good collecting points for scanning bicycles, maybe some rewards if a stolen bicycle is found. Buyers could easily check bicycles if they were reported as stolen (not considering the difficulty of how to establish ownership).
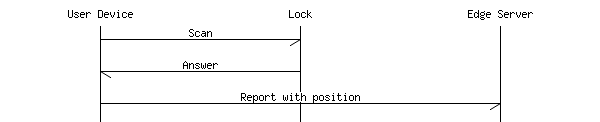 |
Pull architecture |
Technology requirements
The technologies that come to my mind are
Barcode,
QR-Code,
play some humanly not hearable noise and decode in an app,
NFC,
ZigBee,
6LoWPAN,
Bluetooth,
Bluetooth Smart,
GSM,
UMTS,
LTE,
NB-IOT. Next I will look at the main differentiation/constraints of these technologies and provide a small explanation and finish how these constraints interact with each other.
World wide usable
Radio Technology operates on a specific set of radio frequencies (Bands). Each country may manage these frequencies separately and this can lead to having to use the same technology on different bands depending on the current country. This will increase the complexity of the antenna design (or require multiple of them), make mechanical design more complex, makes software testing more difficult, production testing, etc. Or there might be multiple users/technologies on the same band (e.g. wifi + bluetooth or just too many wifis).
Power consumption
Each radio technology requires to broadcast and might require to listen or permanently monitor the air for incoming messages (“paging”). With NFC the scanner might be able to power the device but for other technologies this is unlikely to be true. One will need to define the lifetime of the device and the size of the battery or look into ways of replacing/recycling batteries or to charge them.
Range
Different technologies were designed to work with sender/receiver being away at different min/max. distances (and speeds but that is not relevant for the lock nor is the bandwidth for our application). E.g. with Near Field Communication (NFC) the workable range is meters while with GSM it will be many kilometers and with UMTS the cell size depends on how many phones are currently using it (the cell is breathing).
Pick two of three
Ideally we want something that works over long distances, requires no battery to send/receive and the system is still pushing out the position/acceleration/event report to servers. Sadly this is not how reality works and we will have to set priorities.
The more bands to support, the more complicated the antenna design, production, calibration, testing. It might be that one technology does not work in all countries or that it is not equally popular or the market situation is different, e.g. some cities have city wide public hotspots, some don’t.
Higher power transmission increases the range but increases the power consumption even more. More current will be used during transmission which requires a better hardware design to buffer the spikes, a bigger battery and ultimately a way to charge or efficiently replace batteries.Given these constraints it is time to explore some technologies. I will use the one already mentioned at the beginning of this section.
Technologies
Technology | Bands | Global coverage | Range | Battery needed | Scan Device needed | Cost of device | Arch. | Comment |
---|
Barcode/QR-Code | Optical | Yes | Centimeters | No | App scanning barcode required | extremely low | Pull | Sticker needs to be hard to remove and visible, maybe embedded to the frame |
Play audio | Non human hearable audio | Yes | Centimeters | Yes | App recording audio | moderate | Pull | Button to play audio? |
NFC | 13.56 Mhz | Yes | Centimeters | No | Yes | extremely low | Pull | Privacy issues |
RFID | Many | Yes, but not on single band | Centimeters to meters | Yes | Receiver required | low | Pull | Many bands, specific readers needed |
Bluetooth LE | 2.4 Ghz | Yes | Meters | Yes | Yes, but common | low | Pull/Push | Competes with Wifi for spectrum |
ZigBee | Multiple | Yes, but not on single band | Meters | Yes | Yes | mid | Push | Not commonly deployed, software more involved |
6LoWPAN | Like ZigBee | Like ZigBee | Meters | Yes | Yes | low | Push | Uses ZigBee physical layer and then IPv6. Requires 6LoWPAN to Internet translation |
GSM | 800/900, 1800/1900 | Almost besides South Korea, Japan, some islands | Kilometers | Yes | No | moderate | Push | Almost global coverage, direct communication with backend possible |
UMTS | Many | Less than GSM but South Korea, Japan | Meters to Kilometers depends on usage | Yes | No | high | Push | Higher power usage than GSM, higher device cost |
LTE | Many | Less than GSM | Designed for kilometers | Yes | No | high | Push | Expensive, higher power consumption |
NB-IOT (LTE) | Many | Not deployed | Kilometers | Yes | No | high | Push | Not deployed and coming in the future. Can embed GSM equally well into a LTE carrier |
Conclusion
Both a push and pull architecture seem to be feasible and create different challenges and possibilities. A pull architecture will require at least Smartphone App support and maybe a custom receiver device. It will only work in regions with lots of users and making privacy/tracking more difficult is something to solve.
For push technology using GSM is a good approach. If coverage in South Korea or Japan is required a mix of GSM/UMTS might be an option. NB-IOT seems nice but right now it is not deployed and it is not clear if a module will require less power than a GSM module. NB-IOT might only be in the interest of basestation vendors (the future will tell). Using GSM/UMTS brings its own set of problems on the device side but that is for other posts.